
What is ES6?
ECMAScript 6, also known as ES6 and ECMAScript 2015, was the second major revision to JavaScript.
ES6 official name is ECMAScript 6 but ECMAScript 6 huge people call ES6.
ES6 refers to version 6 of the ECMA Script programming language. ECMA Script is the standardized name for JavaScript and version 6 is the next version after version 5.
ES6 brings new feature :
1 Const Keyword :
The const keyword allows you to declare a constant.
const value cannot be changed.

try to change const value output show error “assignment to constant variable”.
2 Let Keyword:
The Let keyword allows you to declare a variable with block scope.
let keyword like var keyword but some different.

let keyword value change possible only same block scope.
3 Template strings:
Template literals are string literals allowing embedded expressions. You can use multi-line strings and string interpolation features with them.
They were called “template strings” in prior editions of the ES2015 specification.
Template string are enclosed by the backtick (` `).
Example:


4Arrow functions :
Arrow functions were introduced in ES6.
Arrow functions allow us to write shorter function syntax


5 Destructuring Assignment :
The destructuring assignment syntax is a JavaScript expression that makes it possible to unpack values from arrays, or properties from objects, into distinct variables.
Example


6 Error Handling
Try …Catch
The try statement allows you to define a block of code to be tested for errors while it is being executed.
The catch statement allows you to define a block of code to be executed, if an error occurs in the try block.
try … catch handle run time errors.

this code error finds the 2nd line so the 3rd line not run.
referanceError x is not define.
try … catch format:
try{
// code test
}catch(){
// Error Handle
}
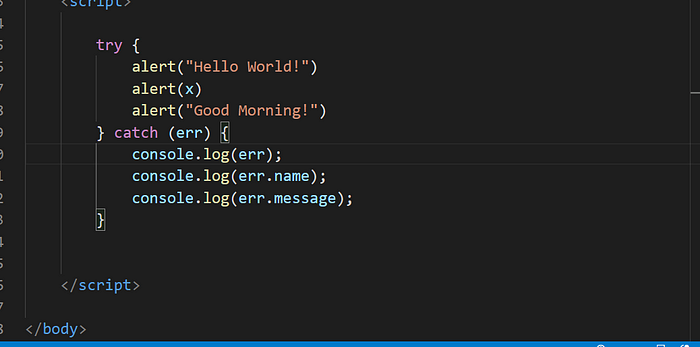
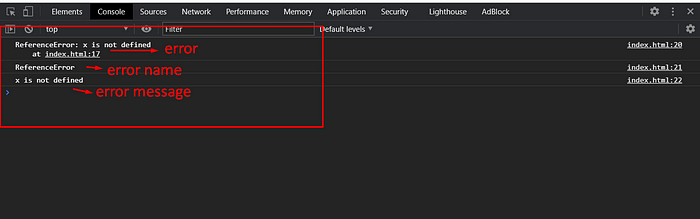
7 Default Parameter :
The default parameter is a way to set default values for function parameters a value is no passed in (ie. it is undefined
).
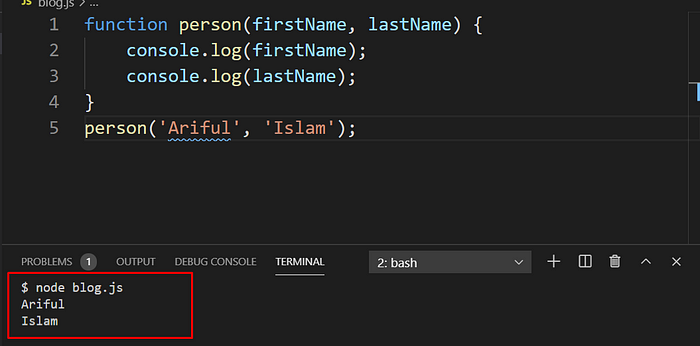

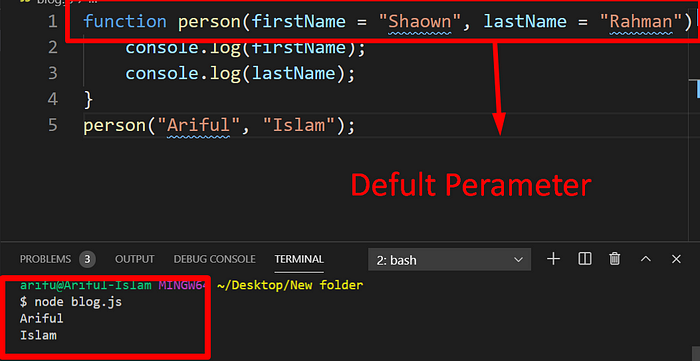
if person() not write perameter then defult perameter work.
8 Comments
JavaScript comments can be used to explain JavaScript code, and to make it more readable.
JavaScript comments can also be used to prevent execution, when testing alternative code.
Single-Line Comments:
single-line comments start “ // ”



9 Coding Style
Our code must be as clean and easy to read as possible.
That is actually the art of programming — to take a complex task and code it in a way that is both correct and human-readable. A good code style greatly assists in that.
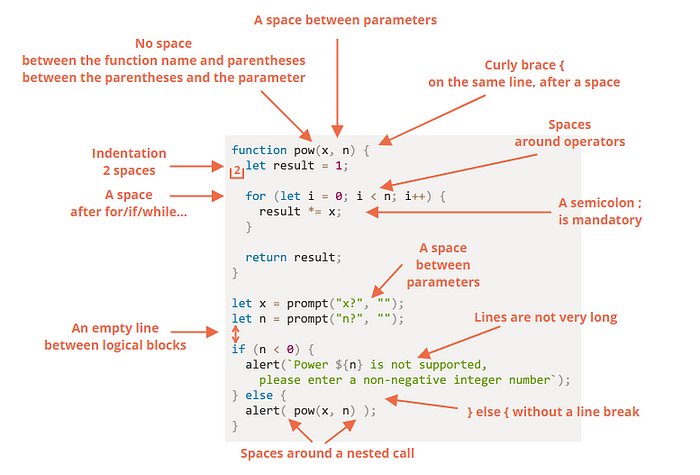
Beginners sometimes do that. Bad! Curly braces are not needed:
if (n < 0) {alert(`Power ${n} is not supported`);}
Split to a separate line without braces. Never do that, easy to make an error when adding new lines:
if (n < 0) alert(`Power ${n} is not supported`);
One line without braces — acceptable, if it’s short:
if (n < 0) alert(`Power ${n} is not supported`);
The best variant:
if (n < 0) {
alert(`Power ${n} is not supported`);
}
10 classes
The constructor()
method is a special method for creating and initializing objects created within a class.
The constructor()
method is called automatically when a class is initiated, and it has to have the exact name "constructor", in fact, if you do not have a constructor method, JavaScript will add an invisible and empty constructor method.
Note: A class cannot have more than one constructor() method. This will throw a SyntaxError
.
You can use the super()
method to call the constructor of a parent class (see "More Examples" below).
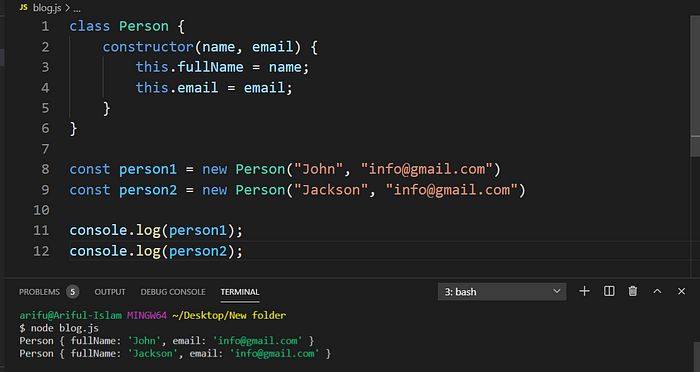